If you ever wanted to integrate CodeIgniter and Zend Framework, you might have come across this tutorial by Daniel Vecchiato.
Whilst Daniel has done a great job demonstrating the possibility of using the two frameworks together, concerns have been made: do we actually need to use hooks?
As I understand it, hooks are used to extend the core functionalities of CodeIgniter (as explained in the user guide). Obviously Zend Framework and CodeIgniter are two different systems and there is no intention for us to extend CodeIgniter’s core functionality with Zend Framework.
Using hooks can be dangerous as it’s system-wide, and it modifies the system behaviour.
What I have done is to simply use CodeIgniter’s library structure to load the Zend Framework resources. Below is the tutorial.
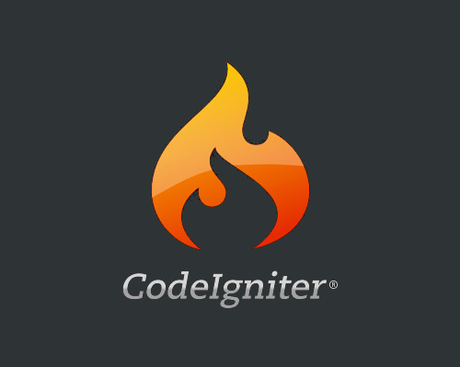
CodeIgniter Framework
Assuming you already have CodeIgniter installed. If not please refer to the user guide for installation.
- Download Zend Framework from the official website.
- Unzip the Zend Framework package, and copy the Zend folder (under Library) to your CodeIgniter installation’s application/libraries/. You can actually place the folder anywhere, but remember to alter the script accordingly (read the comments in the script!).
- Place the library script (provided at the end of the post) in application/libraries/
- Done! That’s all you need to do. Now, let us see an example of using the library.
Usage Sample
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
<?php class Welcome extends Controller { function Welcome() { parent::Controller(); } function index() { $this->load->library('zend', 'Zend/Service/Flickr'); // newer versions of CodeIgniter have updated its loader API slightly, // we can no longer pass parameters to our library constructors // therefore, we should load the library like this: // $this->load->library('zend'); // $this->zend->load('Zend/Service/Flickr'); $flickr = new Zend_Service_Flickr('YOUR_FLICKR_API_KEY'); $results = $flickr->tagSearch('php'); foreach ($results as $result) { echo $result->title '<br />'; } //$this->load->view('welcome_message'); } } ?>
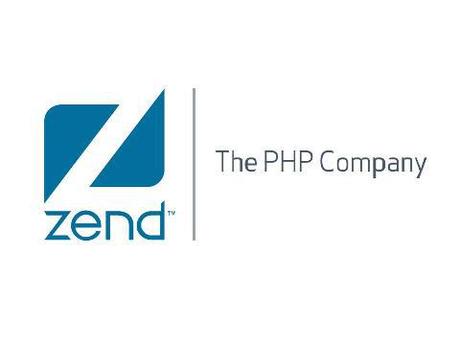
Zend Framework - The PHP Company
Library Script
Copy the code and paste it to a new file called Zend.php in application/libraries/.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
<php if (!defined('BASEPATH')) { exit('No direct script access allowed'); } /** * Zend Framework Loader * * Put the 'Zend' folder (unpacked from the Zend Framework package, under 'Library') * in CI installation's 'application/libraries' folder * You can put it elsewhere but remember to alter the script accordingly * * Usage: * 1) $this->load->library('zend', 'Zend/Package/Name'); * or * 2) $this->load->library('zend'); * then $this->zend->load('Zend/Package/Name'); * * * the second usage is useful for autoloading the Zend Framework library * * Zend/Package/Name does not need the '.php' at the end */ class CI_Zend { /** * Constructor * * @param string $class class name */ function __construct($class = NULL) { // include path for Zend Framework // alter it accordingly if you have put the 'Zend' folder elsewhere ini_set('include_path', ini_get('include_path') PATH_SEPARATOR APPPATH 'libraries'); if ($class) { require_once (string) $class EXT; log_message('debug', "Zend Class $class Loaded"); } else { log_message('debug', "Zend Class Initialized"); } } /** * Zend Class Loader * * @param string $class class name */ function load($class) { require_once (string) $class EXT; log_message('debug', "Zend Class $class Loaded"); } } ?>
Happy coding! Oh and don’t forget, Zend Framework is PHP 5 only, so it won’t work on your PHP 4 installation.
Update: Using it with Kohana
Update: The following instructions are deprecated, please follow the updated one here.
Even though Kohana has the ability to load vendor classes, I still find it useful to use the library approach so that loading Zend Framework libraries will be transparent. :)
Usage is exactly the same as in CodeIgniter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
<?php defined('SYSPATH') or die('No direct script access.'); /** * Zend Framework Loader * * Put the 'Zend' folder (unpacked from the Zend Framework package, under 'Library') * in CI installation's 'application/libraries' folder * You can put it elsewhere but remember to alter the script accordingly * * Usage: * 1) $this->load->library('zend', 'Zend/Package/Name'); * or * 2) $this->load->library('zend'); * then $this->zend->load('Zend/Package/Name'); * * * the second usage is useful for autoloading the Zend Framework library * * Zend/Package/Name does not need the '.php' at the end */ class Zend { /** * Constructor * * @param string $class class name */ function __construct($class = NULL) { // include path for Zend Framework // alter it accordingly if you have put the 'Zend' folder elsewhere ini_set('include_path', ini_get('include_path') PATH_SEPARATOR APPPATH 'libraries'); if ($class) { require_once (string) $class EXT; Log::add('debug', "Zend Class $class Loaded"); } else { Log::add('debug', "Zend Class Initialized"); } } /** * Zend Class Loader * * @param string $class class name */ function load($class) { require_once (string) $class EXT; Log::add('debug', "Zend Class $class Loaded"); } } ?>
Source: beyondcoding.com.